views
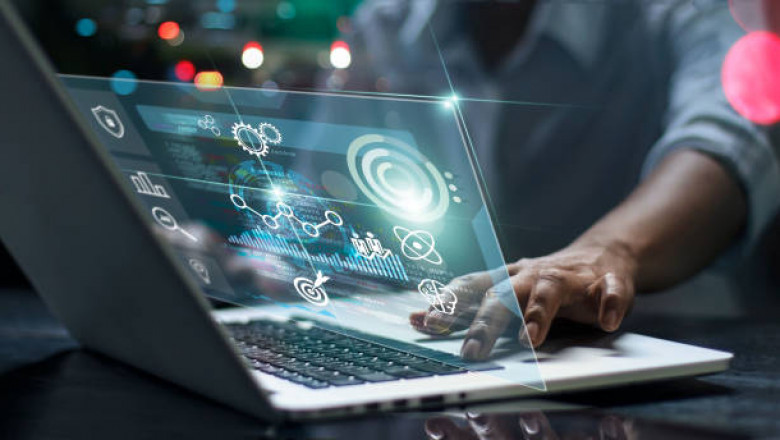
Overview of Data Structures
A computer programme is a set of instructions for carrying out a specific task. A computer program may be required to store, retrieve, and perform computations on the data to accomplish this. A data structure is a named location where data can be stored and organized. We can write efficient and optimized computer programmes by learning data structures and algorithms (DSA).
Bit magic
The "Bit Magic" method allows us to work directly on binary data or bits, which speeds up code execution. Or
By identifying one or more bit patterns or bit numerals, you can increase the effectiveness of the algorithms.
-
A brief overview of the binary and decimal number systems
-
Binary number system to Decimal number system and vice versa conversion.
-
Binary number addition and subtraction.
Bitwise operators
We have Arithmetic operators for working with decimal numbers and Bitwise operators for working with binary numbers.
The Bitwise operators are as follows:
-
Bitwise (AND)
-
Bitwise (OR)
-
Bitwise (XOR)
-
Bitwise (NOT)
-
Right Shift
-
Left Shift
Significance of these operations
-
General application
-
Swapping
-
Even/Odd
For detailed information, explore data structures and algorithms courses and learn these DSA skills.
Recursion and Backtracking
Backtracking is an algorithmic technique for recursively solving problems by attempting to build a solution incrementally, one piece at a time, removing those solutions that fail to satisfy the problem's constraints at any point in time. Backtracking is also an improvement over the brute force approach. So, the idea behind the backtracking technique is to search for a solution to a problem among all possible solutions. Initially, we begin backtracking from one possible option, and if the problem is solved with that option, we return the solution; otherwise, we backtrack and choose another option from the remaining options available.
There may also be cases where none of the options provides a solution, in which case we understand that backtracking will not provide a solution. Backtracking can also be considered a type of recursion. This is because the process of selecting a solution from the available options is repeated recursively until we either don't find a solution or reach the final state. So we can conclude that backtracking at each step eliminates options that cannot provide us with a solution and advance us to options that may provide us with a solution.
Recursion
A recursive algorithm can easily solve some problems. Recursive functions solve specific problems by calling themselves and solving smaller subproblems of the original problem. Many more recursive calls can be generated as and when needed. It is critical to understand that to end the recursion process; we must provide a specific case. As a result, we can state that the function always calls itself a simplified version of the original problem.
Arrays
An array is a collection of contiguous memory locations containing similar elements or data items of the same type. In simple terms, arrays are commonly used in computer programming to organize the same data type.
Hashing
Hashing is the process of converting one value into another based on a given key or string of characters. This is usually represented by a shorter, fixed-length value or key that represents the original string and makes it easier to find or use it.
Strings
A string can be found almost anywhere. You enter a String, i.e. your password, as soon as you log into your device. In fact, this article is made up entirely of Strings. When you come across textual data, it is almost always made up of Strings.
In programming, a String is used as a data type in the same way that an int or float might be; the difference is that a String deals with textual data. It can include letters, numbers, spaces, and special characters. Strings are frequently surrounded by double quotation marks ("This is a String"). Strings can be viewed as a thread connecting various letters.
Linked list
A linked list is a linear collection of data elements whose order is determined by their physical placement in memory. Instead, each element refers to the one before it.
Stacks
A stack is a linear data structure where tasks are carried out in a particular sequence. There are numerous real-life examples of stacks. Consider the stacking of plates in the canteen. The plate at the top is the first to be removed, whereas the plate at the bottom remains in the stack for the longest time.
Dynamic Programming
Dynamic Programming is primarily an improvement on simple recursion. We can use Dynamic Programming to optimize recursive solutions with repeated calls for the same inputs. The idea is to save the results of subproblems, so we don't have to recompute them later. Join the system design course to learn how DSA are used to design websites and applications. The course training offes domain specific knowledge and tools to stay ahead of the competition.